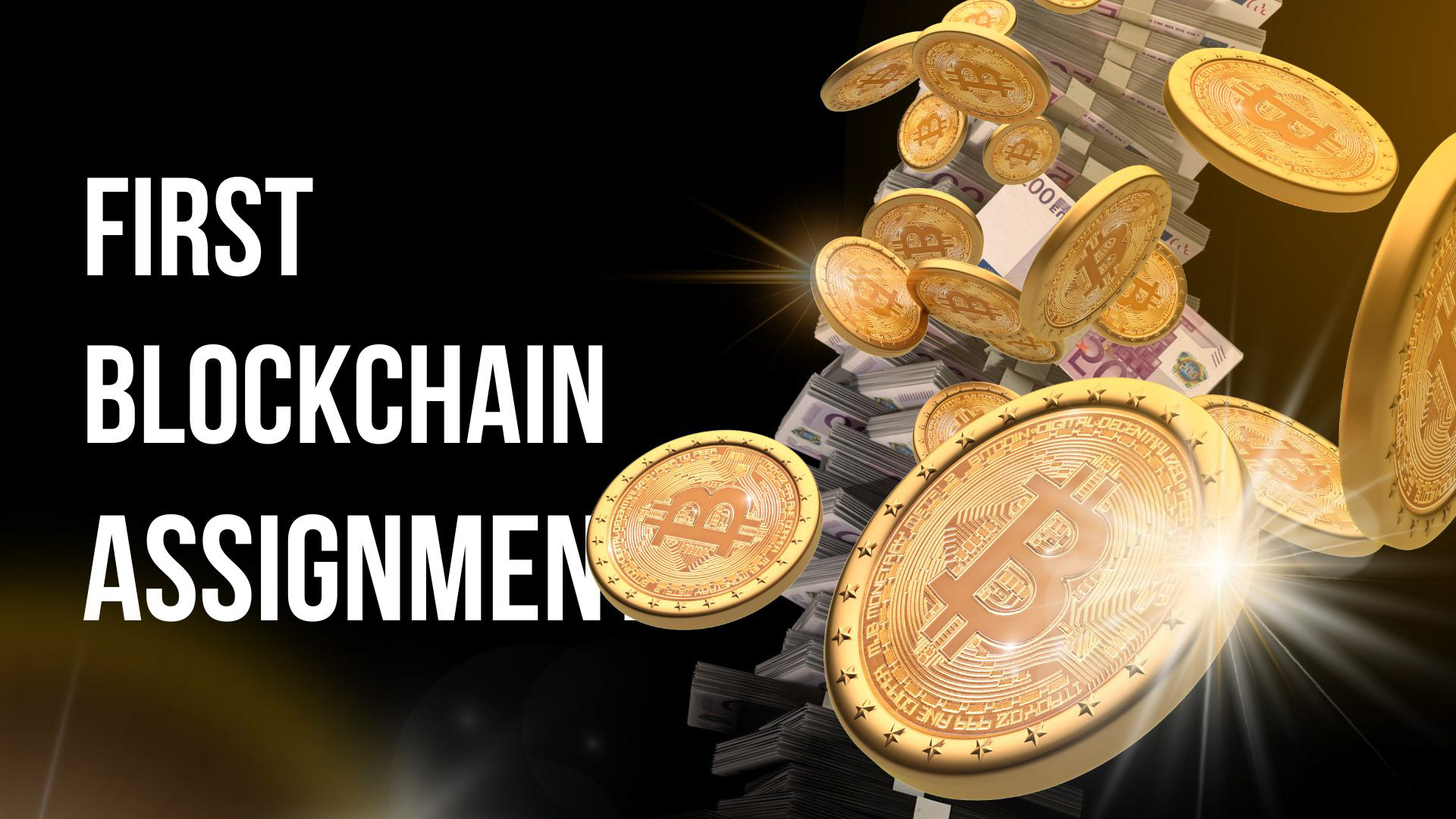
This is a project from the HKU master’s program Fintech elective. It demonstrates how blockchain works using JavaScript by building a simple cryptocurrency system called Mac_Coin. The project includes wallet creation, digital signature with secp256k1, transaction handling, mining, and balance checking. It also shows how blockchain ensures data cannot be changed by validating the integrity of the chain.
こちらは、香港大学(HKU)修士課程のFintech選択科目のプロジェクトです。JavaScriptを使ってブロックチェーンの仕組みを再現し、mac_Coinというシンプルな仮想通貨システムを構築する。ウォレットを生成し、secp256k1で署名を行い、取引を処理し、マイニングを実行し、残高を確認する機能を実装する。また、チェーンの整合性を検証することで、ブロックチェーンが改ざんに強いことを示す。
Creating a blockchain
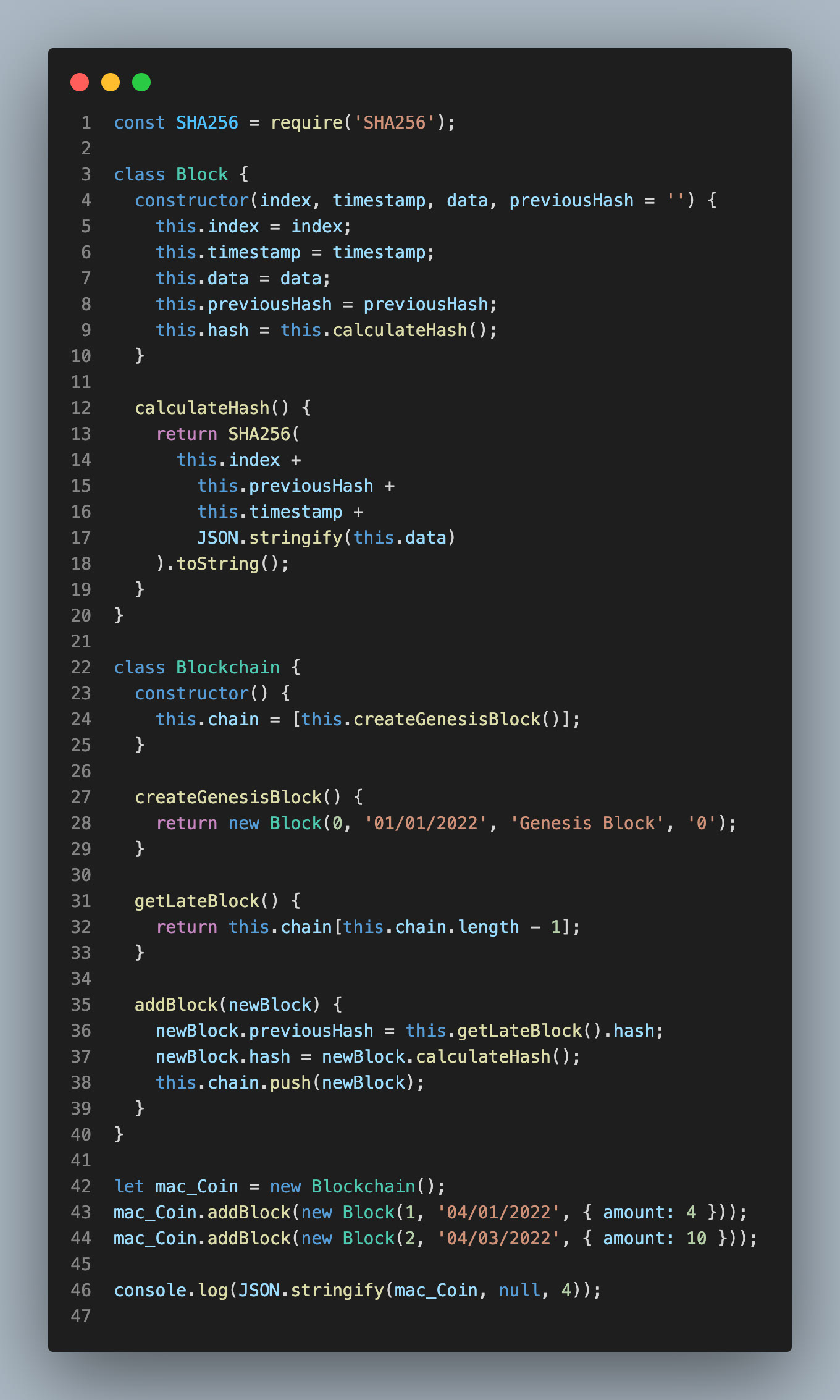
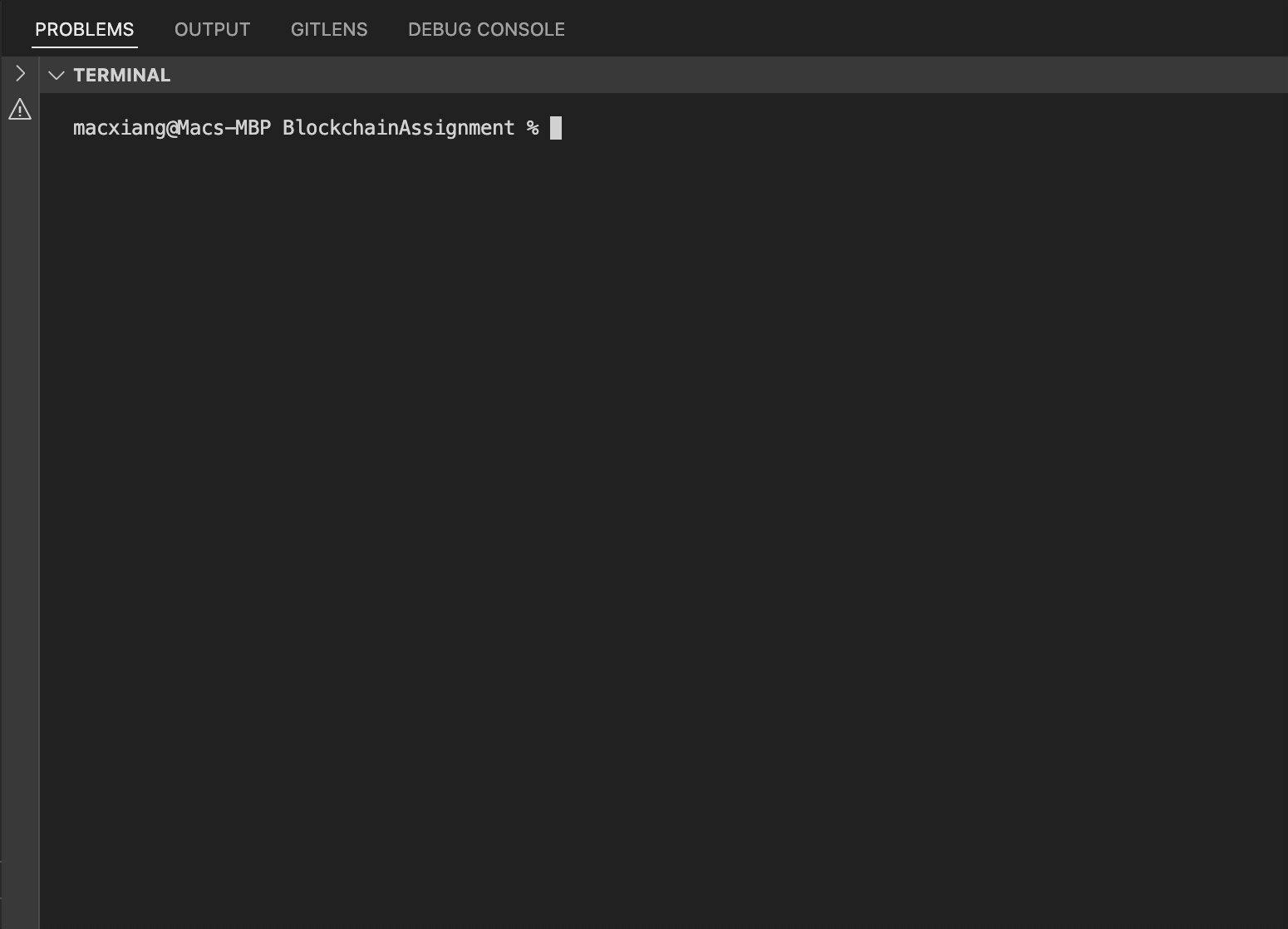
The first step is creating the basic structure of a blockchain using JavaScript. It includes a Block class to store index, timestamp, data, and hash, and a Blockchain class to manage the chain. Each block’s hash is calculated using SHA256, and new blocks are linked to the previous one using its hash. The chain starts with a Genesis Block and grows by adding new blocks with transaction data.
最初のステップは、JavaScriptでブロックチェーンの基本構造を作ること。Blockクラスでインデックス、タイムスタンプ、データ、ハッシュを管理し、Blockchainクラスでブロック同士をつなげていく。各ブロックのハッシュはSHA256で生成され、前のブロックのハッシュを使って安全につながれていく。チェーンはジェネシスブロックから始まり、トランザクションデータを含む新しいブロックを追加することで成長する。
Verify the blockchain
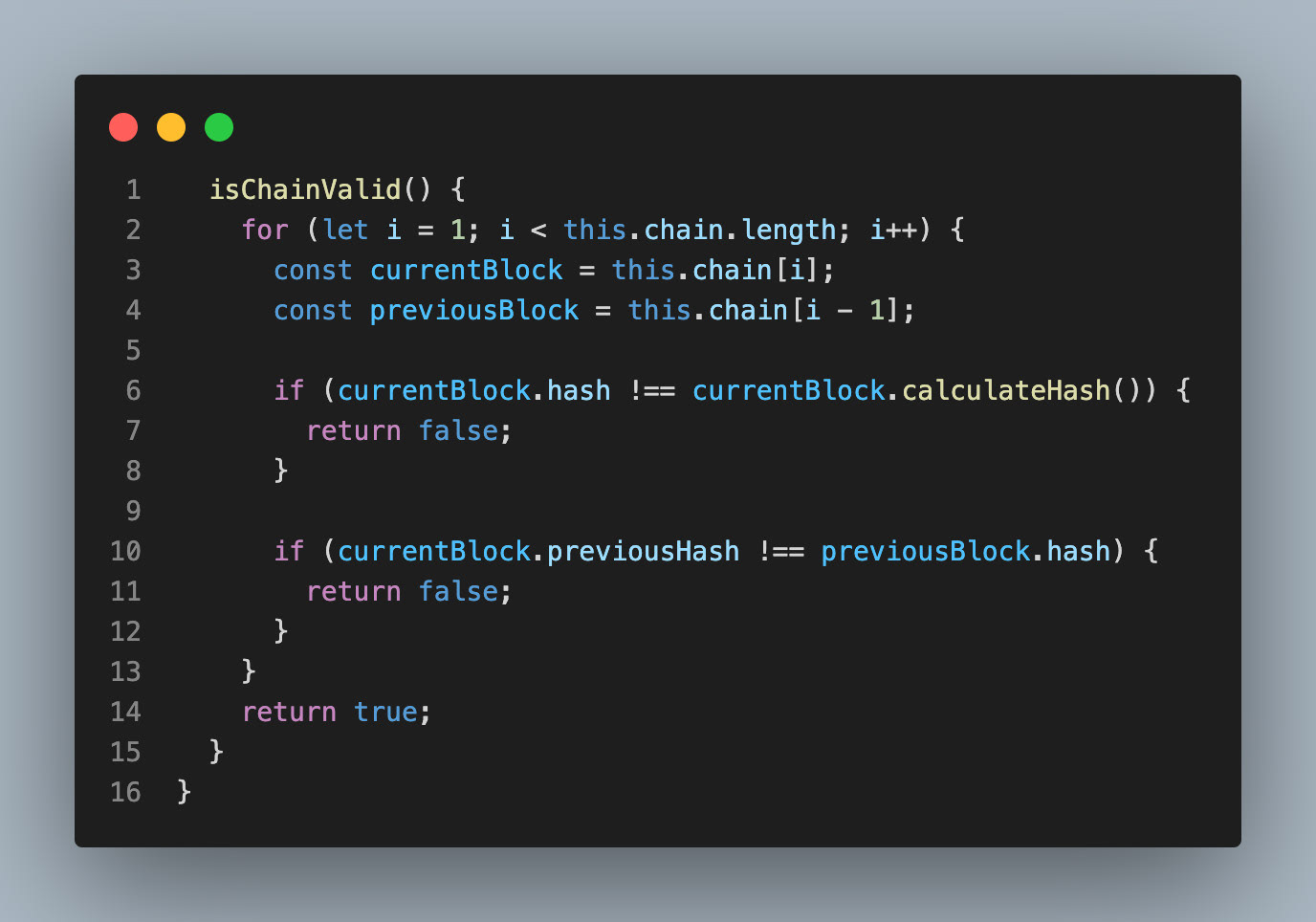
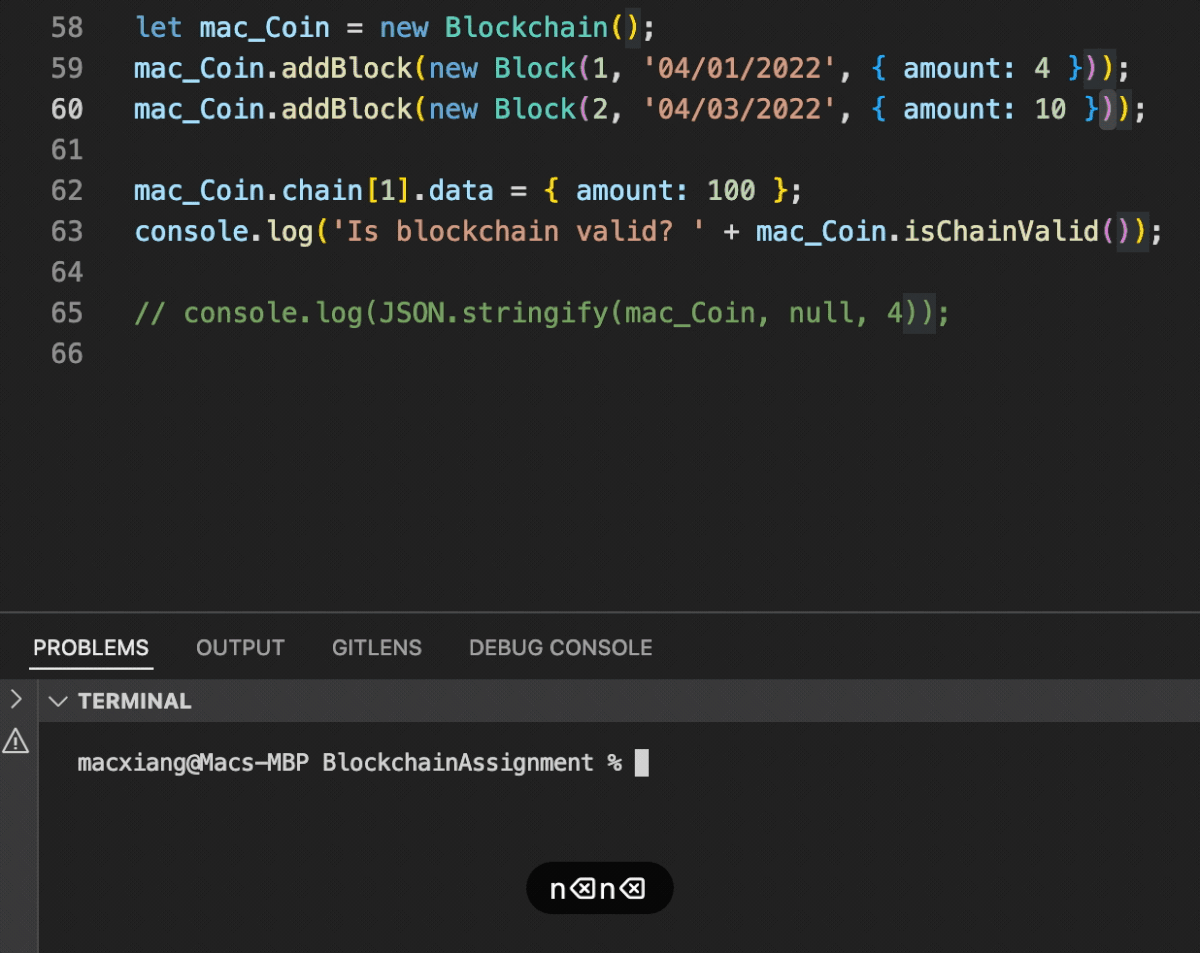
The next step is to verify the integrity of the blockchain. The isChainValid() function goes through each block and checks two things: whether the block’s hash is still valid, and whether it correctly links to the previous block. If either check fails, it means the chain has been tampered with, and the function returns false. This step ensures the blockchain remains secure and trustworthy.
次のステップは、ブロックチェーンの整合性を確認すること。isChainValid()関数では、各ブロックのハッシュが正しいか、前のブロックと正しくつながっているかをチェックする。どちらかに問題があればfalseを返し、チェーンに改ざんがあったことがわかる。この処理によって、ブロックチェーンの安全性と信頼性が保たれる。
Proof-of-Work
Mining - to prove that you have put a lot of computing power into making a block
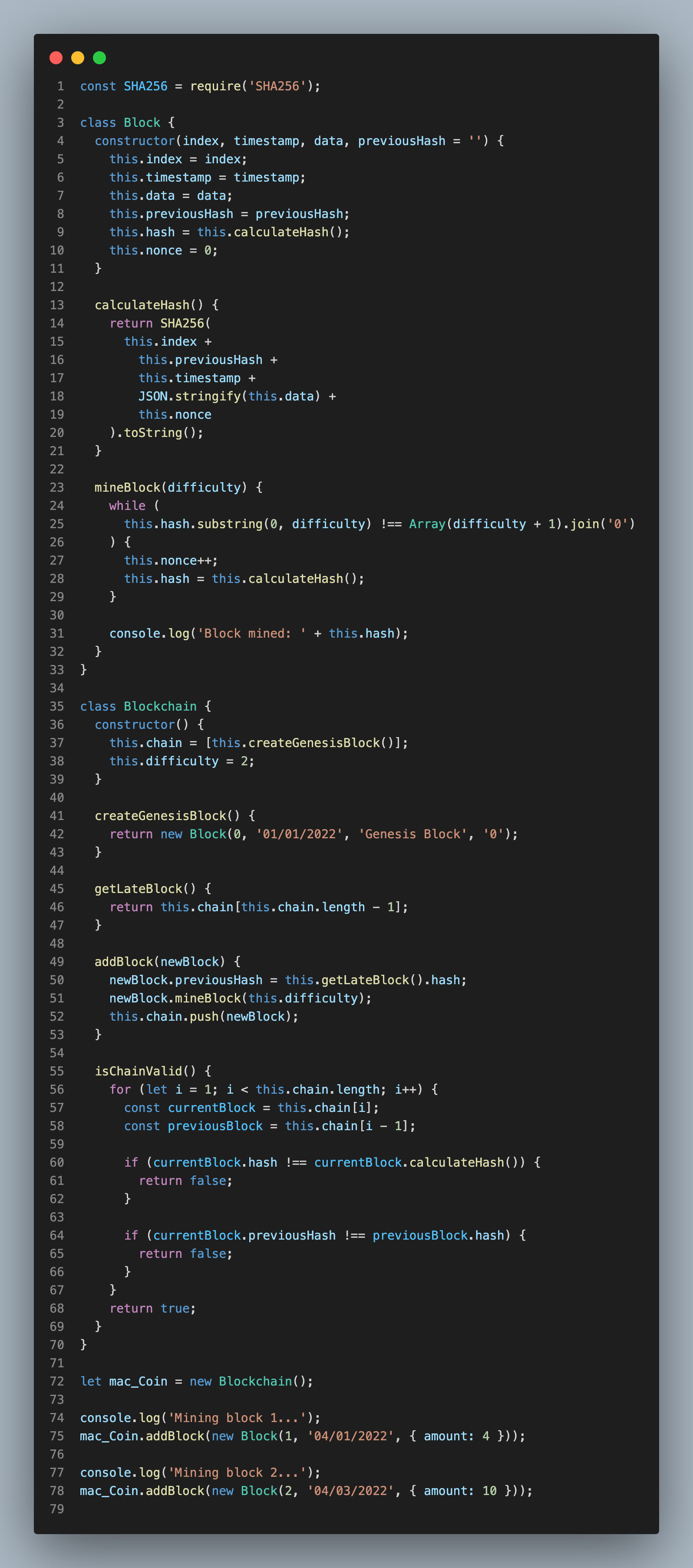
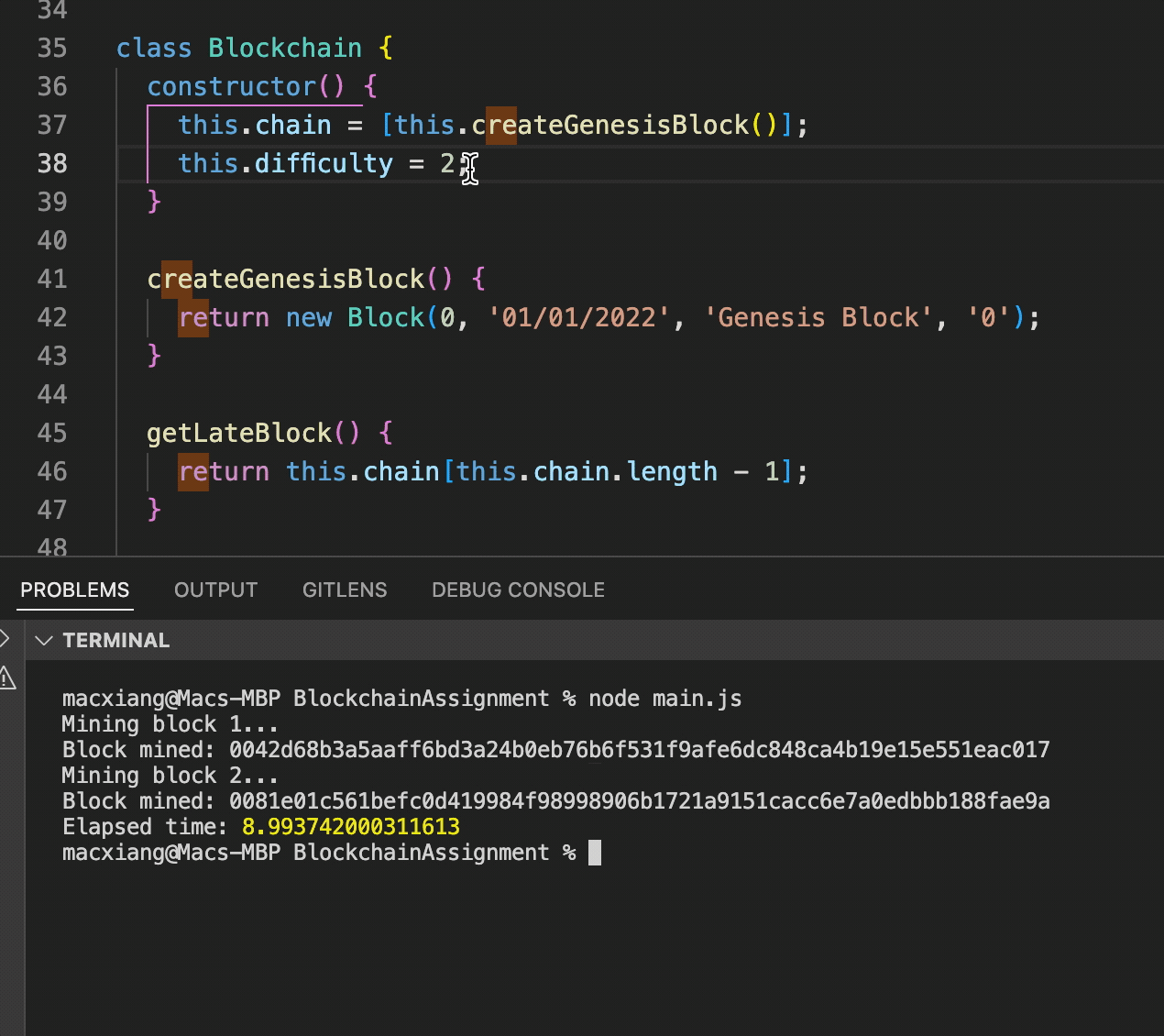
Proof-of-Work is implemented through a mining process where each block must solve a computational puzzle. The block’s hash must begin with a specific number of zeros, defined by the difficulty level. To achieve this, the code keeps changing the nonce value and recalculating the hash until it meets the condition. This ensures that creating a block requires real computational effort, preventing spam or tampering.
Proof-of-Workはマイニングによって実装されている。各ブロックは、難易度で指定された数のゼロから始まるハッシュを見つける必要がある。そのために、コードではnonceの値を何度も変えながらハッシュを再計算する。これにより、ブロックの作成には実際に計算コストがかかり、不正やスパムを防ぐことができる。Mining rewards & transactions
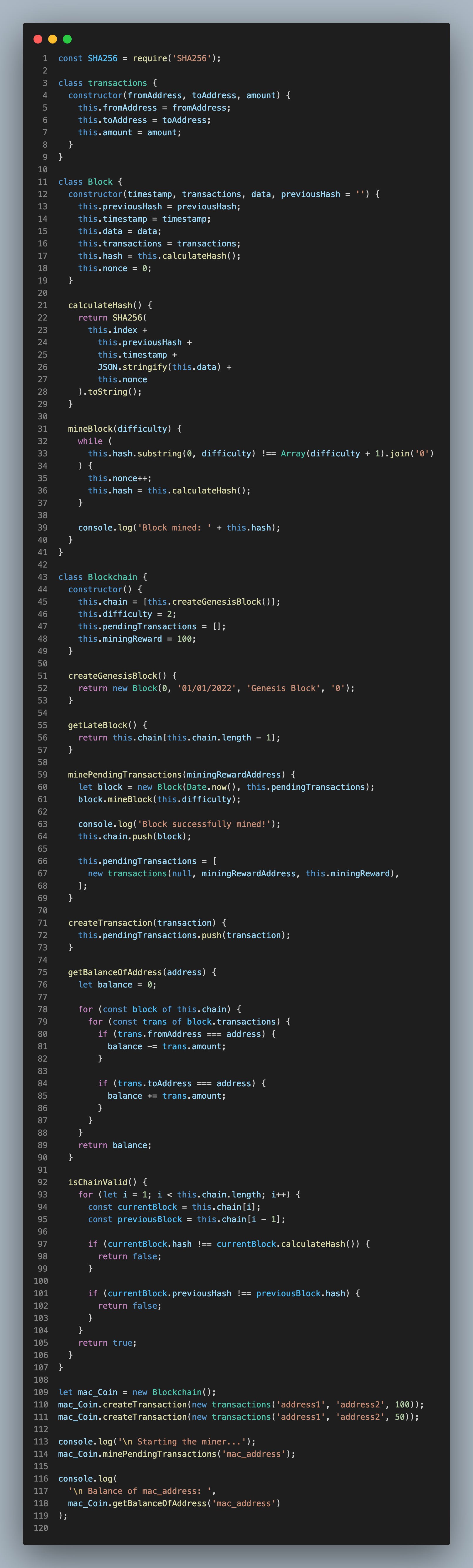
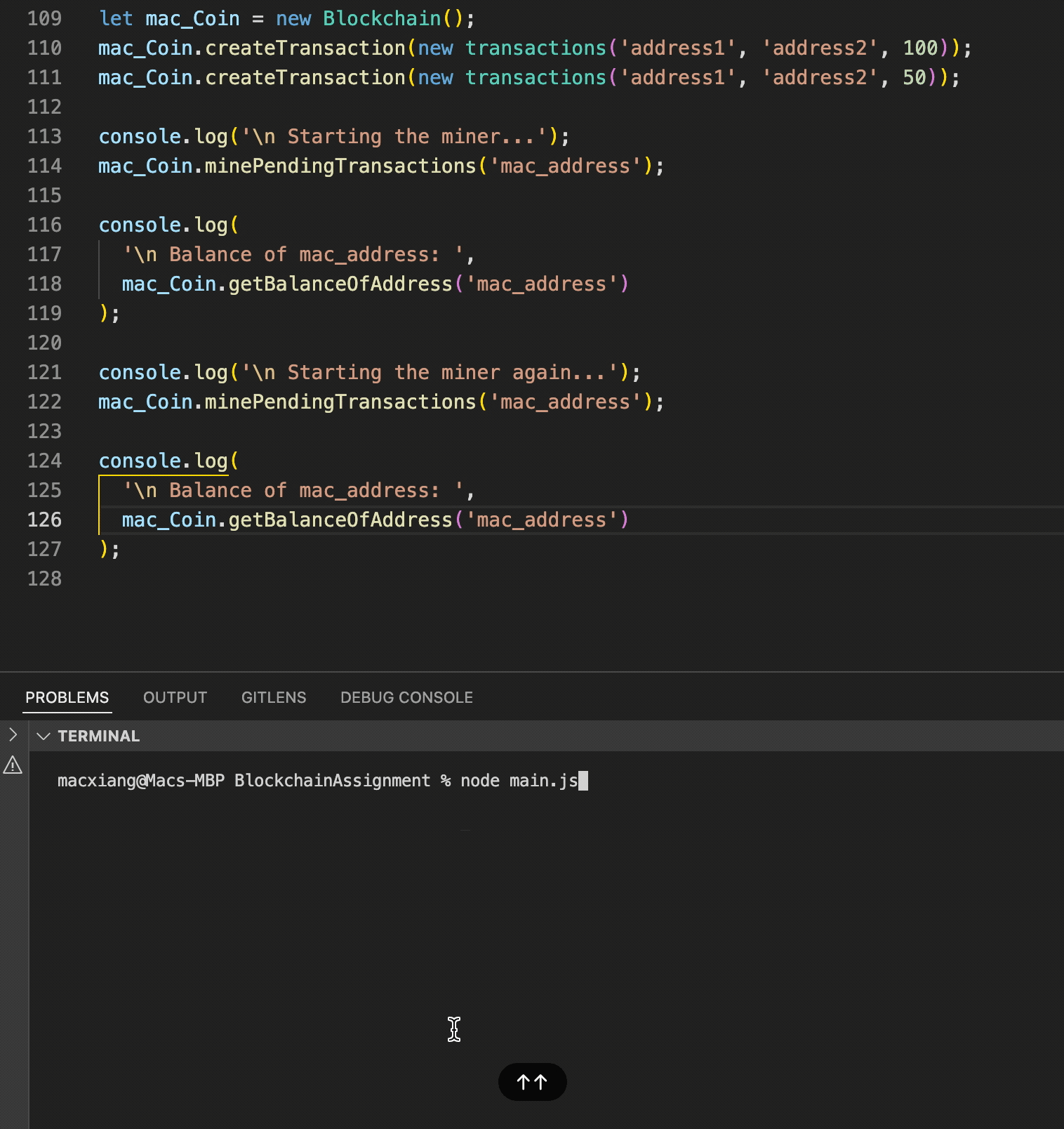
Transactions are created by sending coins from one address to another and are stored as pending until they are added to a block. When mining occurs, all pending transactions are bundled into a new block and added to the chain. As a reward, the miner automatically receives a fixed amount of coins (here, 100) through a special transaction with a null sender. This simulates how real blockchain systems like Bitcoin reward miners for securing the network.
取引は送金元と送金先、金額を指定して作成され、ブロックに追加されるまでpendingTransactionsに保存される。マイニングの際に、それらの取引がまとめられて新しいブロックとしてチェーンに追加される。マイナーには報酬として、送信元がnullの特別なトランザクションで100コインが自動的に付与される。これは、ビットコインのような実際のブロックチェーンがマイナーに報酬を与える仕組みを再現している。
Key Generator
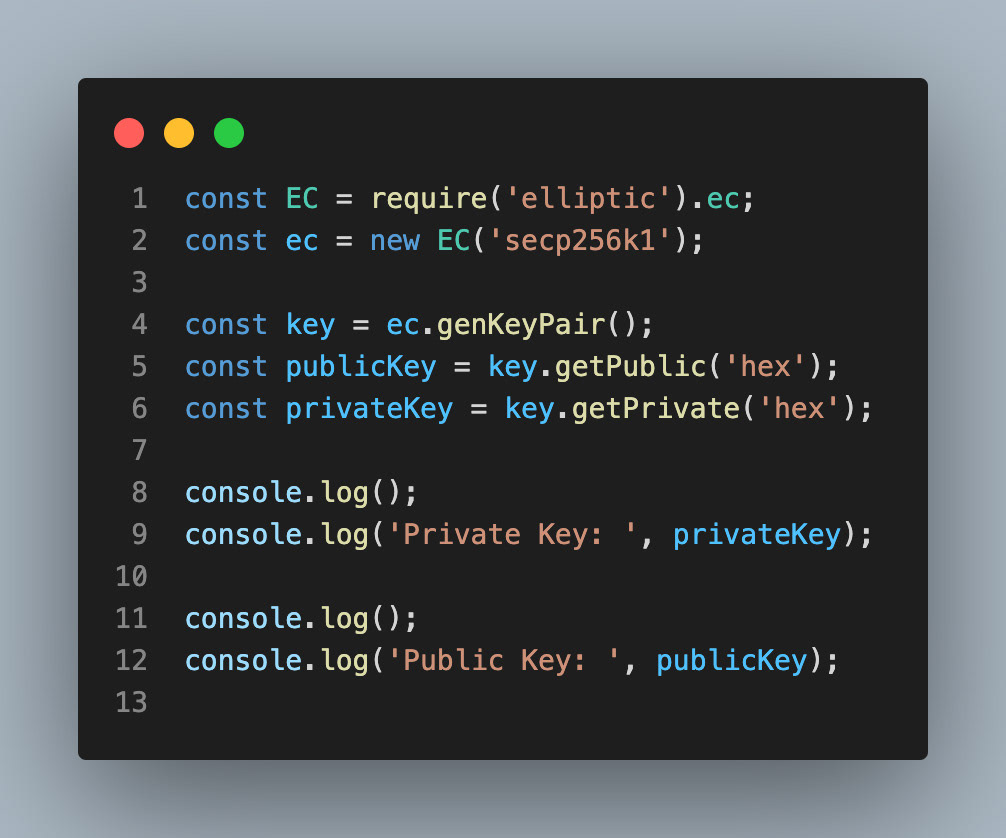
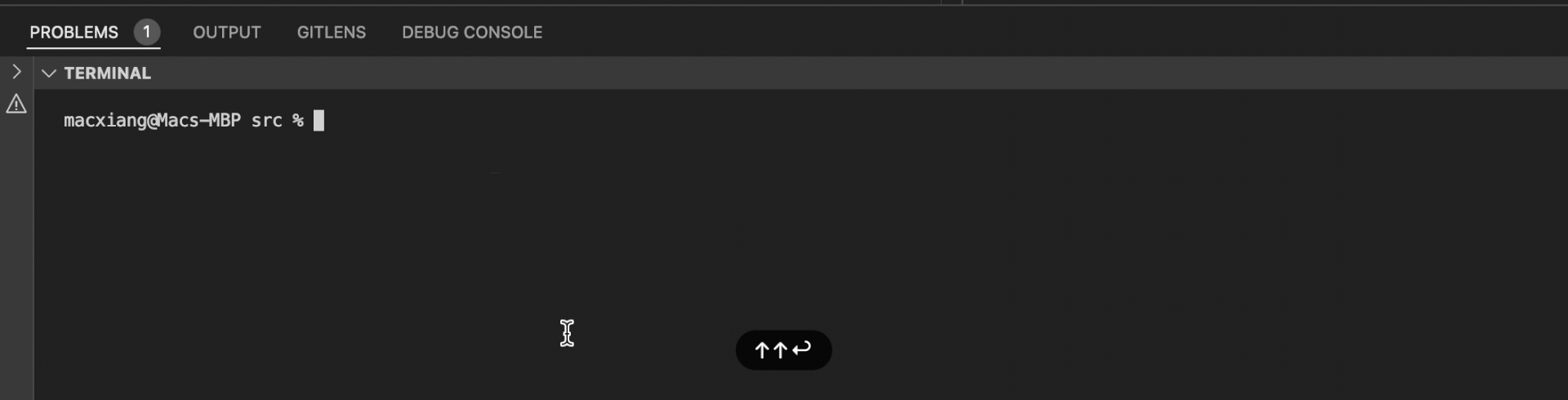
To securely manage ownership and authorize transactions, a key pair is generated using elliptic curve cryptography (secp256k1). The private key is used to sign transactions, while the public key functions as the wallet address. This cryptographic setup ensures that only the rightful owner can send funds, adding a crucial layer of trust and security to the blockchain system.
取引の所有権と認証を安全に管理するために、楕円曲線暗号(secp256k1)を用いて鍵ペアを生成する。秘密鍵は取引に署名するために使い、公開鍵はウォレットアドレスとして機能する。この暗号技術によって、正当な所有者だけが送金できる仕組みが成立し、ブロックチェーン全体の信頼性とセキュリティが高まる。
Mining rewards & transactions
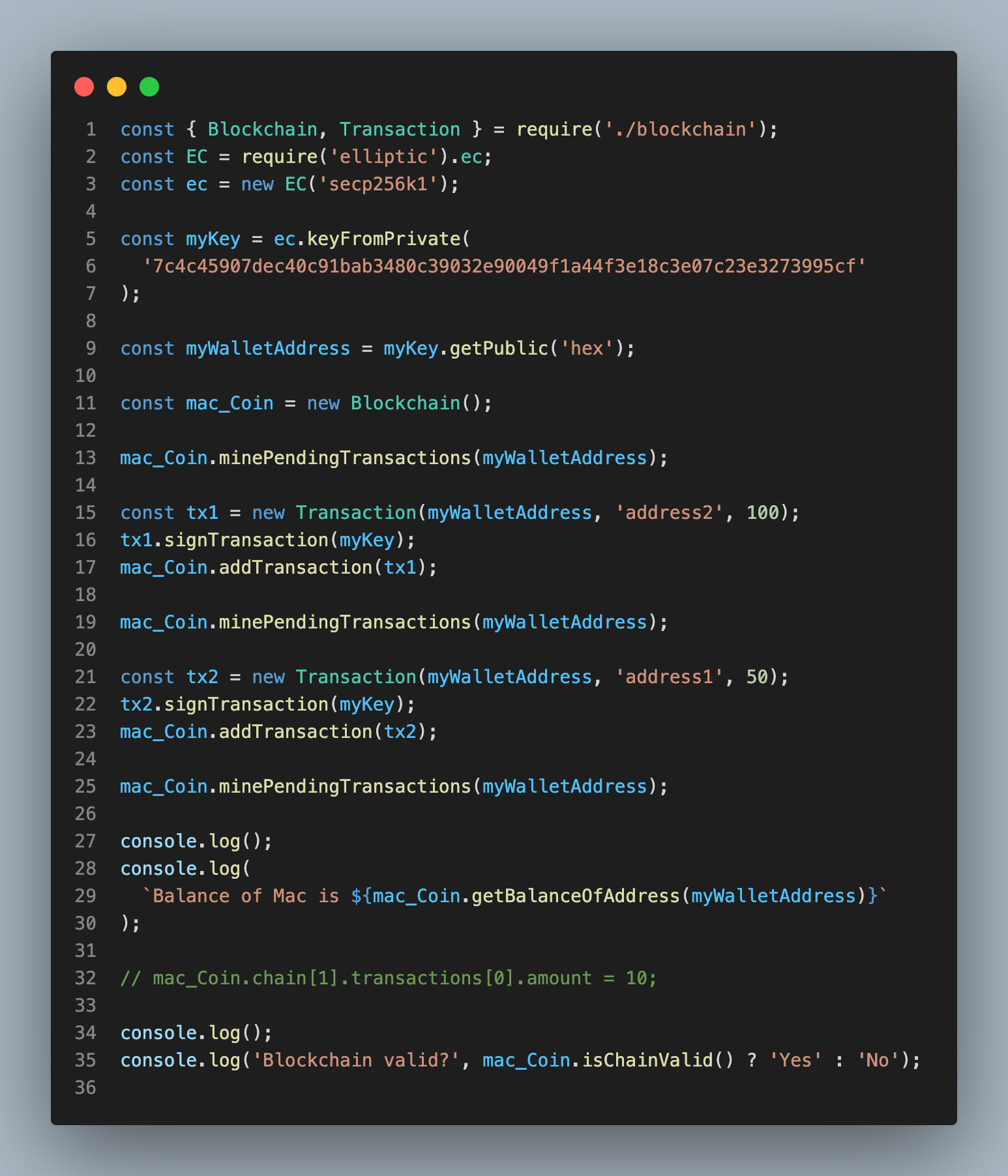
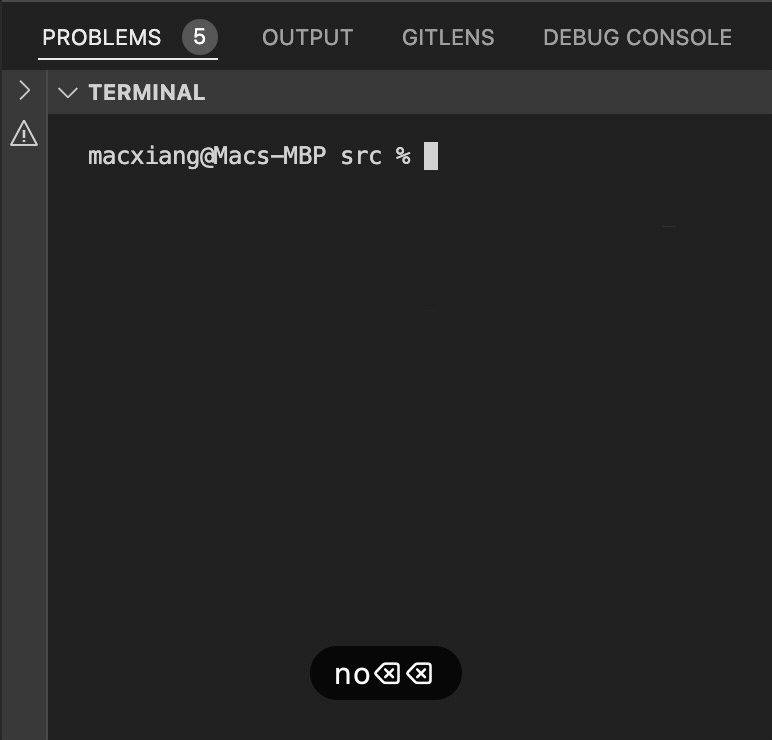
Transactions are created by specifying a sender, receiver, and amount, and are signed using the sender’s private key to ensure authenticity. These signed transactions are collected and added to the blockchain through mining. When a block is successfully mined, the miner receives a reward sent to their wallet address. This mechanism encourages participation and secures the network by requiring computational effort to validate and record transactions.
取引は送信元・送信先・金額を指定して作成され、送信元の秘密鍵で署名することで正当性を保証する。署名された取引はマイニングによってブロックにまとめられ、ブロックチェーンに追加される。ブロックが正常にマイニングされると、マイナーには報酬がウォレットアドレス宛に支払われる。この仕組みにより、ネットワークの安全性が保たれ、マイニングへの参加が促進される。
View the entire [source code] here